--オリジナルのオブジェクトを継承するには--
【開発環境】
OS;Window10
Webブラウザ:Google Chrome
テキストエディタ:Brackets
【ポイント】
・object.setPrototypeOf()メソッドを使用してプロトタイプチェーンを接続すると、既存のオブジェクトを継承できる。
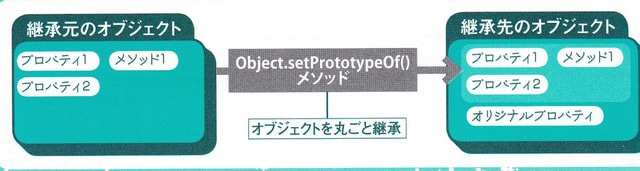
・Call()メソッドを使用すると、新たなオブジェクトコンストラクタ内で、継承元のコンストラクタを呼び出せる。

◆既存のオブジェクトを継承する
Javascriptでは、新たなオブジェクト(子オブジェクト)のプロトタイプチェーンを、継承元のオブジェクト(新オブジェクト)に接続することで、継承出来る。
そのためには、setPrototypeOf()メソッドを使用する。
・Customerを継承するGoldCustomerを作成してみる


・プログラム
<!DOCTYPE html>
<html lang="ja">
<head>
<meta>
<title>JavaScriptのテスト</title>
</head>
<body>
<script>
// Customerコンストラクタ
function Customer(num, name) {
// プロパティ
this.number = num;
this.name = name;
}
// Customerに表示メソッドを追加
Customer.prototype.showInfo = function () {
console.log("番号:", this.number," 名前:", this.name);
};
// GoldCustomerコンストラクタ;Customerの継承
function GoldCustomer(num, name, birthDate) {
// Cusomerのプロパティ
this.number = num;
this.name = name;
// GoldCusomerのプロパティ←新たに追加
this.birthdate = birthDate;
}
// GoldCustomerがCustomerを継承するにはsetPrototypeOf()メソッドを使う→プロトコルチェーン
Object.setPrototypeOf(GoldCustomer.prototype,Customer.prototype);
// GoldCustomerのインスタンスを生成
var gc1 = new GoldCustomer(15, "田中一郎", new Date(1980, 7, 3));
→三番目の引数で、誕生日としてDateオブジェクトのインスタンスを渡している。
// Customerの表示メソッド
gc1.showInfo();
// GoldCusomerに追加されたbirthdateプロパティを表示
console.log("誕生日:", gc1.birthdate.toLocaleDateString());
</script>
</body>
</html>
実行結果

◆GoldCustomerコンストラクタからCustomerコンストラクタを呼び出すには
・call()メソッドを使うことで、GoldCustomerコンストラクタ内で、再度Customerコンストラクタを定義しなくても良くなる。
・書式
継承元のコンストラクタ.call(this,引数1,引数2);
・サンプルコード
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="utf-8">
<title>JavaScriptのテスト</title>
</head>
<body>
<script>
// Customerコンストラクタ
function Customer(num, name){
// プロパティ
this.number = num;
this.name = name;
}
// Customerにメソッドを追加
Customer.prototype.showInfo = function(){
console.log("番号:", this.number, " 名前:", this.name);
};
// GoldCustomerコンストラクタ
function GoldCustomer(num, name, birthDate){
// Cusomerコンストラクタを呼び出し、再初期化する。thisは自分自身のこと
Customer.call(this, num, name);
// GoldCusomerのプロパティ
this.birthdate = birthDate;
}
// GoldCustomerはCustomerを継承するようにする
Object.setPrototypeOf(GoldCustomer.prototype,Customer.prototype);
// GoldCustomerのインスタンスを生成
var gc1 = new GoldCustomer(15, "田中一郎",new Date(1980, 7, 3));
// Customerのメソッド
gc1.showInfo();
// GoldCusomerのbirthdateプロパティ
console.log("誕生日:", gc1.birthdate.toLocaleDateString());
</script>
</body>
</html>
実行結果
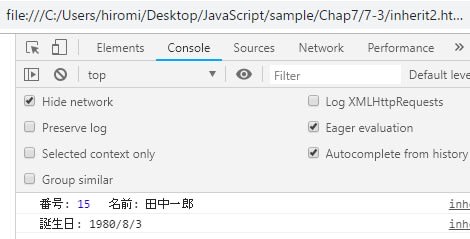
◆GoldCustomerオブジェクトにメソッドを追加するには
prototypeプロパティを使用して、誕生日(birthdateプロパティ)から年齢を求めるgetAge()メソッドを追加する。
例文
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="utf-8">
<title>JavaScriptのテスト</title>
</head>
<body>
<script>
// Customerコンストラクタ
function Customer(num, name) {
// プロパティ
this.number = num;
this.name = name;
}
// Customerにメソッドを追加
Customer.prototype.showInfo = function () {
console.log("番号:", this.number," 名前:", this.name);
};
// GoldCustomerコンストラクタ
function GoldCustomer(num, name, birthDate) {
// Cusomerコンストラクタを呼び出す
Customer.call(this, num, name);
// GoldCusomerのプロパティ
this.birthdate = birthDate;
}
// GoldCutsomerはCustomerを継承する
Object.setPrototypeOf(GoldCustomer.prototype,Customer.prototype);
// GoldCustomerにgetAge()メソッドを追加する
GoldCustomer.prototype.getAge = function () {
var now = new Date();
// 今日と誕生日の年の差を求める
var age = now.getFullYear() - this.birthdate.getFullYear();
// 今年の誕生日
var birthdateThisYear = new Date(now.getFullYear(),this.birthdate.getMonth(),
this.birthdate.getDate());
// 誕生日が過ぎていなければ1を引く
if (now < birthdateThisYear) {
age--;
} return age;
}
// GoldCustomerのインスタンスを生成
var gc1 = new GoldCustomer(15, "田中一郎",new Date(1959, 0, 17));
// Customerのメソッド
gc1.showInfo();
// GoldCusomerのbirthdateプロパティ
console.log("誕生日:", gc1.birthdate.toLocaleDateString());
// GoldCusomerのgetAge()メソッド
console.log("年齢:", gc1.getAge());
</script>
</body>
</html>
実行結果
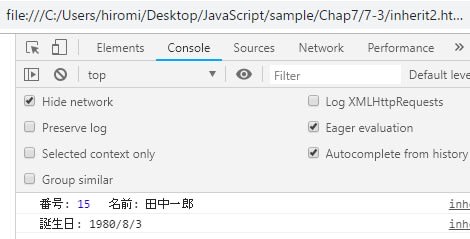
◆Customerオブジェクトにメソッドを追加する
例文
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="utf-8">
<title>JavaScriptのテスト</title>
</head>
<body>
<script>
// Customerコンストラクタ
function Customer(num, name) {
// プロパティ
this.number = num;
this.name = name;
}
// Customerにメソッドを追加
Customer.prototype.showInfo = function () {
console.log("番号:", this.number," 名前:", this.name);
};
// GoldCustomerコンストラクタ
function GoldCustomer(num, name, birthDate) {
// Cusomerコンストラクタを呼び出す
Customer.call(this, num, name);
// GoldCusomerのプロパティ
this.birthdate = birthDate;
}
// GoldCutsomerはCustomerを継承する
Object.setPrototypeOf(GoldCustomer.prototype,Customer.prototype);
// GoldCustomerにgetAge()メソッドを追加する
GoldCustomer.prototype.getAge = function () {
var now = new Date();
// 今日と誕生日の年の差を求める
var age = now.getFullYear() - this.birthdate.getFullYear();
// 今年の誕生日
var bitrhdateThisYear = new Date(now.getFullYear(),
this.birthdate.getMonth(),
this.birthdate.getDate());
// 誕生日が過ぎていなければ1を引く
if (now < bitrhdateThisYear) {
age--;
}return age;
}
// Customerのインスタンスを生成
var c1 = new Customer(5, "伊藤花子");
// GoldCustomerのインスタンスを生成
var gc1 = new GoldCustomer(15, "田中一郎", new Date(1959, 0, 17));
// Customerにメソッドを追加
Customer.prototype.sayHello = function () {
console.log("こんにちは、私は" + this.name + "です。");
}
c1.sayHello();
gc1.sayHello();
</script>
</body>
</html>
・結果
