旧ブログからのコード移植、その3。
Crop は、Mask をつかって、指定色領域を指定の色許容度で透明にする。
SmoothEdge は、Crop された画像のエッジのアルファ値を傾斜をかけて滑らかにする。
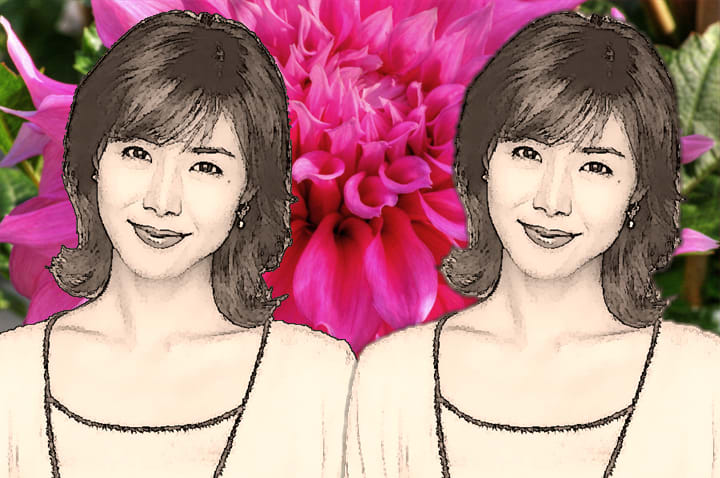
全コードをしめす。
using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Text; using System.Windows.Forms; using System.Drawing.Imaging; using System.Drawing.Drawing2D; using ImageUtils; namespace CropSmoothEdge { public partial class Form1 : Form { public Form1() { InitializeComponent(); } public static bool Crop(ref Bitmap bmp, Bitmap mask, Color maskColor, int tolerance) { if (bmp.PixelFormat != PixelFormat.Format24bppRgb) return false; if (mask.PixelFormat != PixelFormat.Format24bppRgb) return false; if ((tolerance < 0) | (tolerance > 100)) return false; int w = bmp.Width; int h = bmp.Height; if ((w != mask.Width) | (h != mask.Height)) return false; byte redSmall = ImgUtils.AdjustByte(maskColor.R - tolerance); byte redLarge = ImgUtils.AdjustByte(maskColor.R + tolerance); byte greenSmall = ImgUtils.AdjustByte(maskColor.G - tolerance); byte greenLarge = ImgUtils.AdjustByte(maskColor.G + tolerance); byte blueSmall = ImgUtils.AdjustByte(maskColor.B - tolerance); byte blueLarge = ImgUtils.AdjustByte(maskColor.B + tolerance); Bitmap tmp = new Bitmap(w, h, PixelFormat.Format32bppArgb); Graphics g = Graphics.FromImage(tmp); g.DrawImageUnscaled(bmp, 0, 0); g.Dispose(); BmpProc24 src = new BmpProc24(mask); BmpProc32 dst = new BmpProc32(tmp); for (int y = 0; y < h; y++) for (int x = 0; x < w; x++) { src.SetXY(x, y); if ((src.R < redSmall) | (src.R > redLarge) | (src.G < greenSmall) | (src.G > greenLarge) | (src.B < blueSmall) | (src.B > blueLarge)) continue; dst[x, y, eRGB.a] = 0; } ImgUtils.CallDispose(dst, src, bmp); bmp = tmp; return true; } public static bool SmoothEdge(ref Bitmap bmp, int zone) { if (bmp.PixelFormat != PixelFormat.Format32bppArgb) return false; int w = bmp.Width; int h = bmp.Height; Bitmap tmp = bmp.Clone() as Bitmap; ImgUtils.Blur32(ref bmp, zone); BmpProc32 src = new BmpProc32(tmp); BmpProc32 dst = new BmpProc32(bmp); for (int y = 0; y < h; y++) for (int x = 0; x < w; x++) { src.SetXY(x, y); dst.SetXY(x, y); if (dst.A == 0) continue; if (src.A == dst.A) { dst.R = src.R; dst.G = src.G; dst.B = src.B; } } ImgUtils.CallDispose(dst, src, tmp); return true; } private void button1_Click(object sender, EventArgs e) { Bitmap bmp = new Bitmap(@"c:\Home\ImgWork\CropSample.png"); Bitmap mask = new Bitmap(@"c:\Home\ImgWork\CropMask.png"); Bitmap haikei = new Bitmap(@"c:\Home\ImgWork\CropBack.png"); Color maskColor = mask.GetPixel(50, 50); Crop(ref bmp, mask, maskColor, 40); Graphics g = Graphics.FromImage(haikei); g.DrawImage(bmp, -160, 0, bmp.Width, bmp.Height); SmoothEdge(ref bmp, 2); g.DrawImage(bmp, 230, 0, bmp.Width, bmp.Height); g.Dispose(); g = this.CreateGraphics(); g.DrawImageUnscaled(haikei, 5, 35); Clipboard.SetImage(haikei); g.Dispose(); haikei.Dispose(); mask.Dispose(); bmp.Dispose(); } } }
CropSample
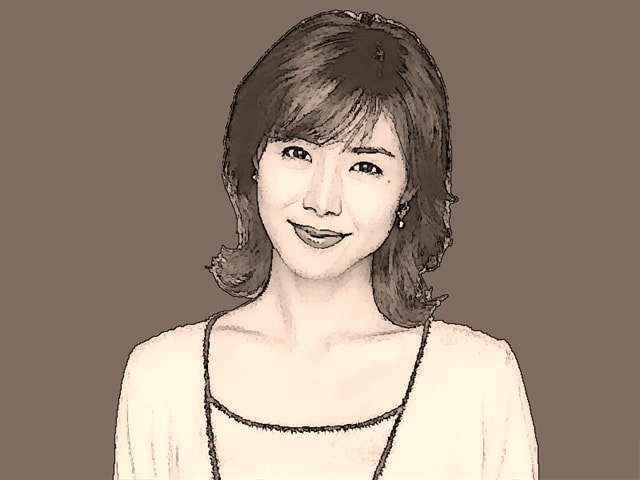
CropMask
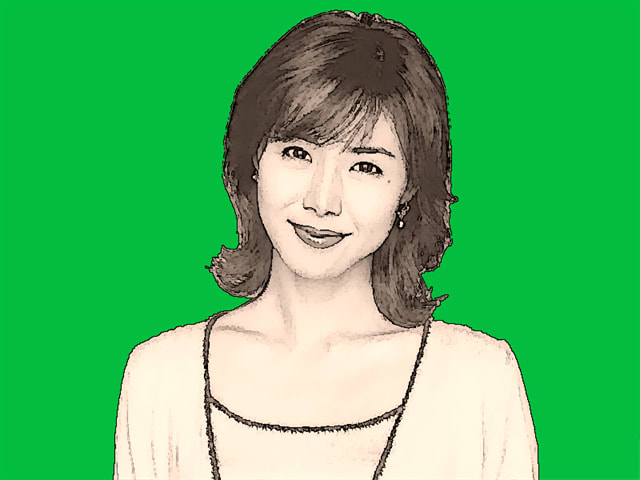
CropBack
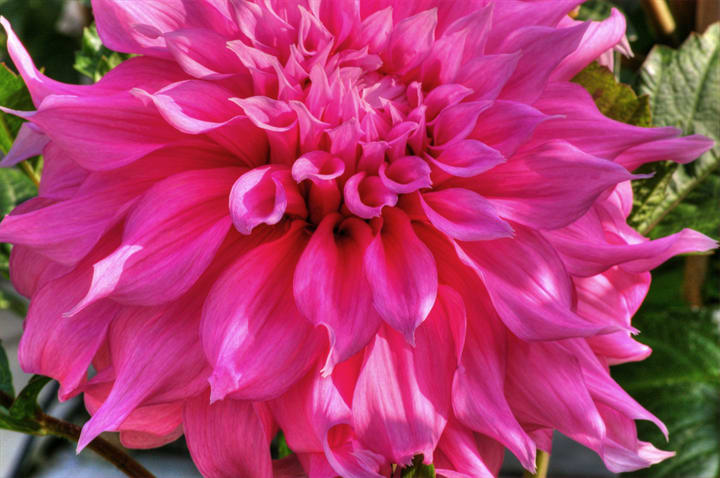